The Riley-O-Matic
by Daniel Iglesia
This project consists of a program that, given a few parameters, generates a piece of music modeled after Terry Riley's "In C", a seminal minimimalist work.
The program generates a SKINI file, which is used in STK to generate the piece.
About Terry Riley's "In C"
The piece consists of 53 musical fragments. A time-keeping instrument (usually piano or marimba) plays an eigth-note pulse. Then, each instrument comes in on the first fragment, and plays it as many times as each individual desires, then moving on the the second fragment, and so on. In all, a hypnotic, polyrhythmic piece occurs.
This link contains the score and the original performance directions.
and here's
an mp3 of the first 5 minutes of a recent recording (played on mallets)(7 MB)
Differences in my implementation, and input parameters
Source Code in C, which I complied on arizona
For my purposes, I changed a few of the performance diretions for my program, and made others variables. My input parameters are:
-t tempo: tempo in bpm. If not specified, the default is a random value from 45 to 135
-r resolution: value 1(boring) to 6. This specifies the smallest note value allowed, as in 1/(2^resolution) is the smallest note value allowed. Default is 4, which allows 16th notes.
-d duration: duration of the piece in measures. default is 100. Maximum is 1000.
-n number of instruments: maximum 20. The first instrument is always the pulse. Default is random number between 5 and 10. Although Riley specified that it could be performed by any number of instruments, this range, I feel, is the most pleasing. Any more and it often sounds very muddy.
Also, Riley wanted the instruments in question to play the written notes, I.E. stay within a small range around middle C. My implementation is different: each instrument is assigned a random octave from 1 to 6 (with a bell curve distribution centered around middle C) to allow for a greater range. This means that fragments are often doubled in different octaves, which sounds cool.
Generation of source material
My implementation, like Riley's, first generates an array of random measures.
To do this, a random integer "density" is created. "Density" is a percent (1-100) probabilty that an event will occur.
The struct "melody" contains just three arrays: "ticker"(which signifies the start of a note), "ender" (signifies the end of the note on that beat, and the incrementation of the index to the pitch array), and "pitches"(many are generated, though not all will be used). The input parameter "resolution" affects a variable "tickpermeasure", which is the number of events that can occur in a measure (for whole notes, it would be 1, for 16th notes, it would be 16). So for each possible event, we assign a "1" with a probabilty of "density".
So here's an example melody with 16 ticks permeasure and a density of 40.
ticker:1 0 1 1 1 1 0 1 0 1 0 0 0 1 0 1
ender:1 1 1 1 1 1 0 0 1 0 0 0 1 0 1 1
pitch:21 17 17 19 17 19 14 17 21 17 24 24 23 21 19 16
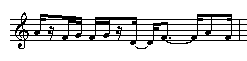
And here's an example with 16 ticks and a density of 10:
ticker:0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0
ender:0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 1
pitch:16 23 21 14 24 24 24 24 24 17 23 12 14 17 12 16
These equates to the following measures of music. The actual pitches are 4 octaves below, but will be transcribed up a certain number of octaves depending upon the assigned range of the instrument that plays it.
Transcription to SKINI
Because I feel the piece sounds best all on the same instrument (and because I didn't feel like tinkering with the source code), I set it up so that the output is all on one SKINI file. The program computes the time, in seconds, between each tick, and prints a counting marker for each tick, then prints all the events that happen at that tick. A sample below shows a measure with the pulse (playing note 60) and another melody. The NoteOff commands with the hash mark are the time keepers.
-NoteOff 0.010000 10 0 0
NoteOn 0.000000 1 60 100
NoteOn 0.000000 2 65 100
-NoteOff 0.150000 10 0 0
NoteOff 0.140000 1 60 100
NoteOff 0.010000 10 0 0
NoteOn 0.000000 1 60 100
NoteOn 0.000000 2 65 100
-NoteOff 0.150000 10 0 0
NoteOn 0.000000 2 65 100
NoteOff 0.140000 1 60 100
-NoteOff 0.010000 10 0 0
NoteOn 0.000000 1 60 100
NoteOn 0.000000 2 65 100
As you can see the time keeper is smart enough to realize if the previous beat had a NoteOff at the end of it or not, and adjust its relative time accordingly.
Picking an STK instrument
Some STK instruments are far better suited to this than others. The instrument should have a large range and not get muddy when combined with other copies of itself. I've tested the intruments and given my opinions:
GOOD:ModalBar (sounds the most like the original), TubeBell (eerie and good),Plucked or StifKarp (a bit bass heavy),BeeThree (very Philip Glassy)
OK: Most of the winds(though they can get muddy: they are usually better at a slower tempo),Blowhole (kind of native american sounding), VoicForm (kind of microtonal, swarm like, Ligeti thing going on here), Moog (envelope is too slow to work at fast tempo), Wurley, Bowed (kind of sedate and quiet).
BAD: Sitar (terrfying swarm of bees), Resonate (static), Shakers (doesn't work, didn't have time to investigate), Mesh2d (non-pitched)
Examples
Example 1
Parameters:
tempo=90
instruments=6
duration=100 measures
resolution=4
The density of each measure is a random number from 10 to 60.
The SKINI file
A chart of the first 6 measures produced
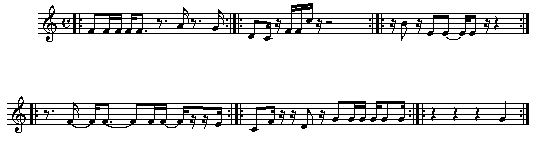
AUDIO FILES:
----played on BeeThree---(pick this one if you must only pick one)
----played on ModalBar---
Example 2
Parameters:
tempo=110
instruments=8
duration=60 measures
resolution=4
The density is fixed at 40.
The SKINI file
A chart of the first 6 measures produced
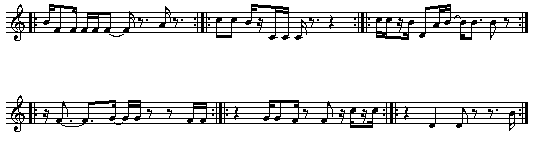
AUDIO FILES:
----played on Plucked ----
----played on TubeBell----
Program Note
Because this whole page outlines the creation of the piece, I won't dwell here. However, In retrospect, some comparison between the original "In C" and my creations is in order. My main though is on the predetermined nature of Riley's measures in comparison to a randomly generated series of measures that has no aesthetic considerations in mind. I feel that though hard to tell the difference at first, the premeditated interlocking of Riley's measures does sound better in the long run.
Original Proposal
I went through a bunch of ideas for this (mostly thinking about vocoders or a new "instrument" that uses a lot of brownian motion with each particle having musical characteristics based on position and velocity), and in the end I would like to return to my primary academic interest of algorithmic composition. I realize this is not quite in line with the goals of this class; however, I plan to integrate it with the Synthesis Toolkit, and hope that this makes it applicable enough.
I have done a few other algorithmic composition projects, namely a Xenakis-style stochastic synthesizer (to which you input a score file of time-based musical "events") ,and a 16th century species counterpoint generator (that I still want to show you sometime). For this, I was inspired by one of the great minimalist pieces, Terry Riley's "In C". The process of performing that piece has definite algorithmic qualities, in which an arbitrary number of musicians each play one of a series of musical figures many times until deciding to move to the next one.
I am currently thinking about trying to create a "minimalist generator" that would create and perform pieces in the style of Terry Riley's "In C" or Steve Reich's "Music for 18 Musicians" (which has a bunch of rythmic groups phasing/fading in and out with each other). Some of the variables are the number of performers and the instrumentation; these could either be specified by the user or developed randomly by the program. Some random chance will be used for when a "musician" move on to the next musical event.
Yet my program could have other, more nuanced features that a traditional musician could not execute with any precision. Imagine a mallet player gradually adding reverb (or phase shift, or tremelo, or varying spectrum shape, or any number of other effects). I have not quite decided how this will be done; I still must work with STK a bit more to figure out how to do this by hacking that code/using SKINI/developing a new score file format.
References
-Technical correspondence with Perry Cook and Ari Lazier
-Information on Terry Riley's "In C" from Experimental Music, by Michael Nyman